Dropdown with Image
You can use an image or Avatar component with `ImgDropdown`.
Examples
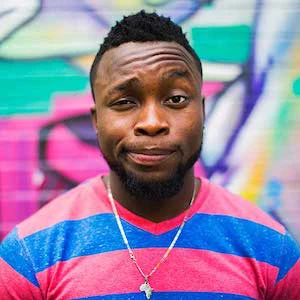
<script>
import { ImgDropdown, Avatar } from 'flowbite-svelte';
let avatar = {
src: '/images/profile-picture-1.webp',
alt: 'My avatar',
size: 12,
border: true,
round: true
};
let items = [
{
href: '/',
name: 'Dashboard'
},
{
href: '/',
name: 'Settings'
},
{
href: '/',
name: 'Sign out'
}
];
</script>
Dropdown position right
You can change the dropdown position to the right by adjusting the `dropdownClass` and add a divider.
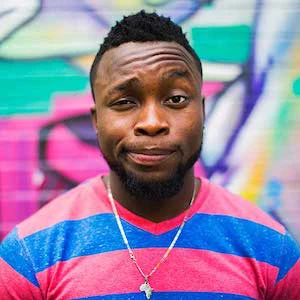
<script>
...
let items2 = [
{
href: '/',
name: 'Dashboard',
divider:true
},
{
href: '/',
name: 'Settings'
},
{
href: '/',
name: 'Sign out'
}
];
let dropdownClass = 'hidden absolute left-10 top-14 z-10 w-44 text-base list-none bg-white rounded divide-y divide-gray-100 shadow dark:bg-gray-700';
</script>
<ImgDropdown items={items2} {dropdownClass}>
<Avatar {avatar} />
</ImgDropdown>
Dropdown position left
Let's move the dropdown to left and add a header.
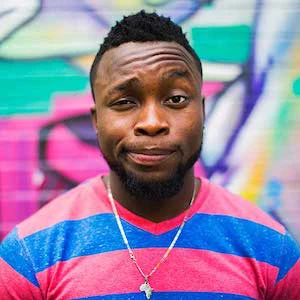
<script>
let dropdownClass2 ='absolute top-14 z-10 w-44 text-base list-none bg-white rounded divide-y divide-gray-100 shadow dark:bg-gray-700';
</script>
<ImgDropdown {items} divClass="relative flex justify-end" >
<div slot="header">
<span class="block text-sm">Bonnie Green</span>
<span class="block text-sm font-medium truncat">name@flowbite.com</span>
</div>
<Avatar {avatar} />
</ImgDropdown>
You can use an image.
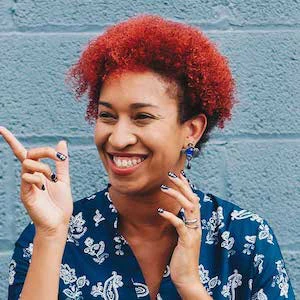
<ImgDropdown {items}>
<img src="/images/profile-picture-3.webp" width='50' alt="something"/>
</ImgDropdown>
Props
The component has the following props, type, and default values. See types page for type information.
Name | Type | Default |
---|---|---|
items | DropdownType[] | |
divClass | string | 'relative' |
dropdownClass | string | 'hidden absolute -left-16 top-14 z-10 w-44 text-base list-none bg-white rounded divide-y divide-gray-100 shadow dark:bg-gray-700' |
headerClass | string | 'py-3 px-4 text-gray-900 dark:text-white' |
linkClass | string | 'block py-2 px-4 text-sm text-gray-700 hover:bg-gray-100 dark:hover:bg-gray-600 dark:text-gray-200 dark:hover:text-white' |